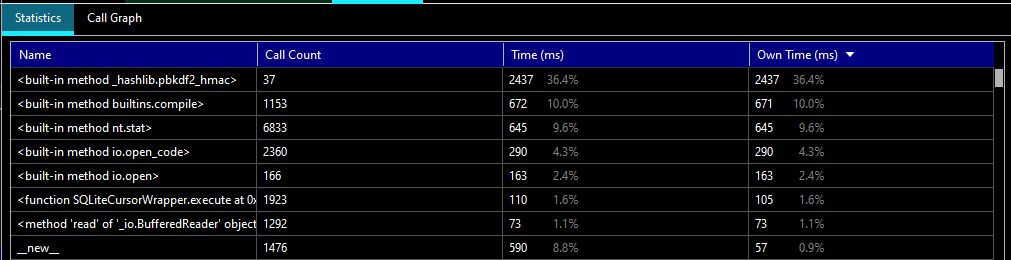
More than a third of the time was taken with a hashlib function. My current testing regime doesn’t take long (about 3 seconds on my slow machine) but any iteration time is precious when you are working on your side project.
Before I wax poetic, here’s the changes you’d make to your project’s settings.py
# This is only needed if you don't already have a PASSWORD_HASHERS list in your settings PASSWORD_HASHERS = [ 'django.contrib.auth.hashers.PBKDF2PasswordHasher', 'django.contrib.auth.hashers.PBKDF2SHA1PasswordHasher', 'django.contrib.auth.hashers.Argon2PasswordHasher', 'django.contrib.auth.hashers.BCryptSHA256PasswordHasher', ] # DO NOT USE INSECURE HASHING OUTSIDE OF DEBUG MODE OR YOU WILL GET HACKED # All of your data will be stolen and all of your good works undone # Avoid having your company added to this list https://haveibeenpwned.com/ if DEBUG: PASSWORD_HASHERS.insert(0, 'django.contrib.auth.hashers.MD5PasswordHasher')
So what’s happening here?
Context
If you’re fairly new to Django you might not know the settings.py file controls the general configuration of your application, and defines arbitrary values available from the settings module (a really useful feature!)
The DEBUG value is set for test environments (earlier in the file) based on environment variables that I control, if you want to learn more check out the DEBUG documentation.
Most values that could exist in your settings file have sane defaults, but it can be a bit confusing that not everything is there at once. If you dont have a PASSWORD_HASHERS list in your settings Django will pick whatever the “right” option is.
In this case we’re defining the standard items and then inserting a new default hash option in the list (during DEBUG mode only.)
This sets PASSWORD_HASHERS to reference a very fast and very weak (full list here).
Using the PyCharm test UI I found my slow machine testing went from 2987ms to 526ms, and improvement of >5x!
The observer effect is in play for the statistics but we still show the entire hashing process gone from the stats:
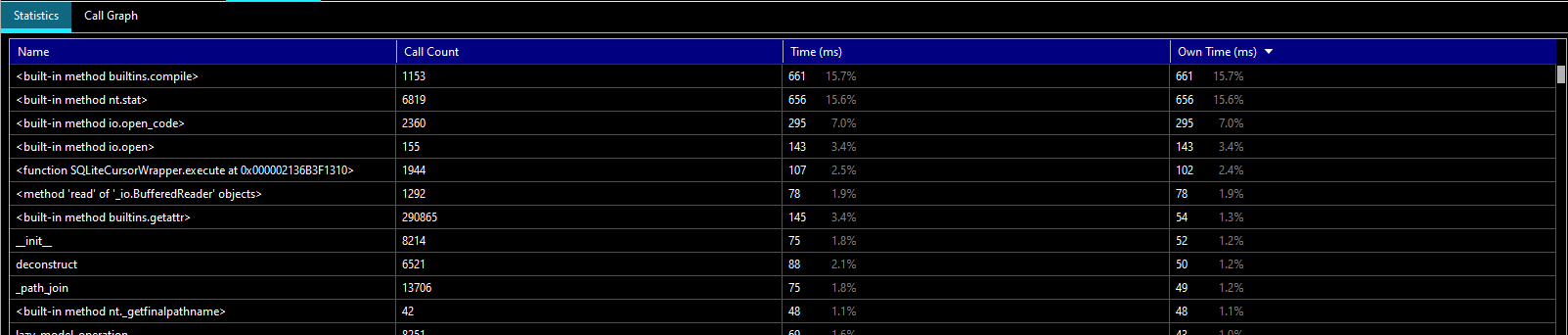
It’s worth repeating – don’t run insecure hashing such as md5 algorithms in production ever. It’s the difference between your password being cracked in seconds and making it impractical for decades or centuries.
It may seem weird, but being purposefully slow is an important feature of cryptographic hashes that you should not attempt to defeat.
If you want to learn more about how cryptographic hash functions work check out Practical Cryptography For Developers.